Changing date formats is a common task in web development, and when working with React.js, it’s essential to understand how to manipulate and present dates effectively. In this article, you will learn how to change date formats in React JS
This skill comes in handy when displaying dates in a user-friendly format or when interacting with APIs that provide date information. In this article, we’ll explore a straightforward guide on how students can change date formats in React.js.
const d = new Date();
let text = d.toString();
output: Sun Feb 04 2024 09:33:32 GMT+0530 (India Standard Time)
const d = new Date();
let text = d.toLocaleString();
output: 2/4/2024, 9:34:52 AM
const dateTime = currentDate.toLocaleString('en-US', { year: 'numeric', month: 'long', day: 'numeric', hour: 'numeric', minute: 'numeric', second: 'numeric' });
console.log(dateTime);
// Output: February 4, 2024, 3:26:45 PM
As React JS is a javascript library we can make use of the Date() object in javascript to change date formats in React JS
Create Date() Object
Start by creating a JavaScript Date
object. You can do this by instantiating a new Date
one or by getting a date from an API response or user input.
const mydate = new Date();
Use toLocaleString
Method:
The toLocaleString
can be used for formatting dates in React as well as javascript. It takes two arguments: the locale (country-specific language ) and an options object. The locale is optional, but it can be useful if you want to display the date in a specific language or region.
const myFormatedDate = mydate.toLocaleString('en-US', { year: 'numeric', month: 'long', day: 'numeric' });
you can adjust the options in the toLocaleString
method according to your required format. You can customize the format by including or excluding options like 'year'
, 'month'
, 'day'
, 'hour'
, 'minute'
, and so on.
NPM packages for formatting Dates
Although we can format date with javascript date object it provides limited options, if you need to format other than date object methods you can use the npm package to format date in react js.
date-format
date-format is a popular npm package to format date. It has 4,323,138 weekly downloads with an unpacked size of 19.7 kB only.
npm i date-format
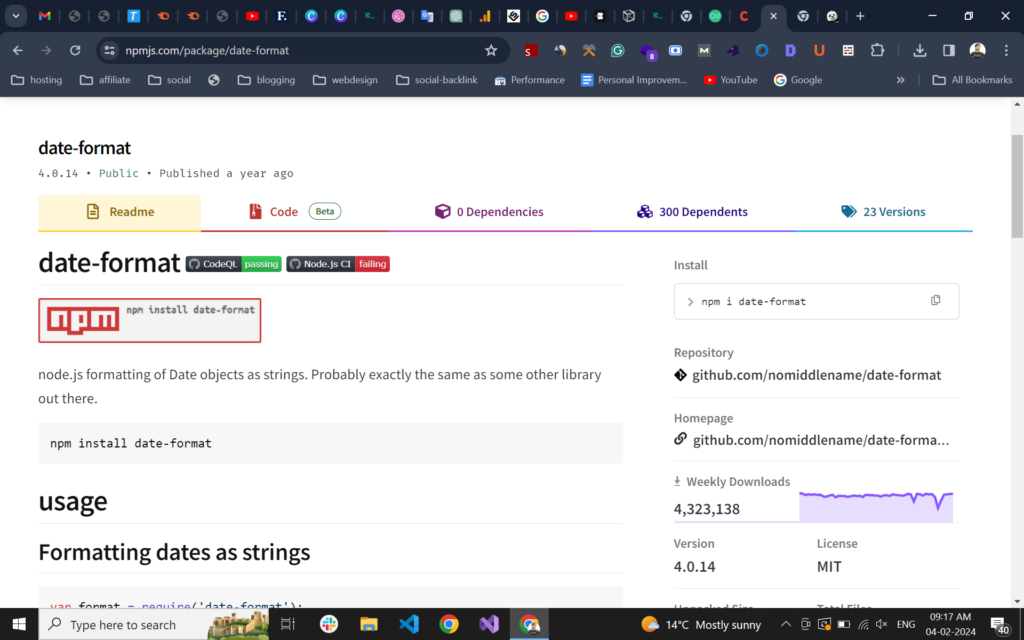
Moment.js
Moment JS is a super popular date format package among JS developers. Mostly all JS developers use this package whether it be jQuery, javascript, or React JS development.
I can provide you with all possible formatting methods for your date format needs.
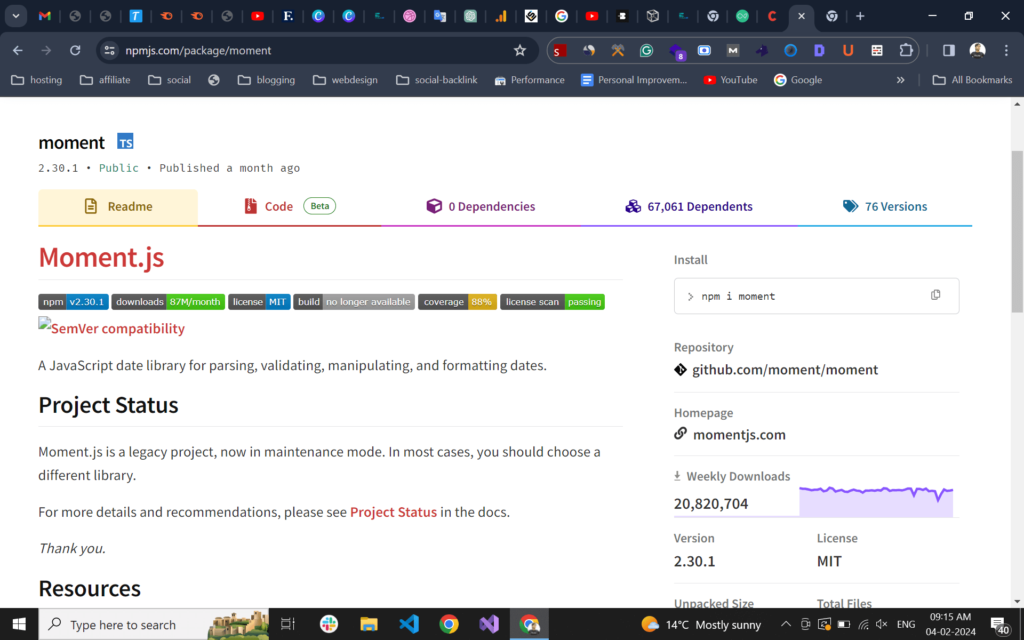
So these were the ways to change date formats in React JS. You utilize the above methods to change the date in different formats.